
- Trending Categories

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary

Different Forms of Assignment Statements in Python
Assignment statement in python is the statement used to assign the value to the specified variable. The value assigned to the variable can be of any data type supported by python programming language such as integer, string, float Boolean, list, tuple, dictionary, set etc.
Types of assignment statements
The different types of assignment statements are as follows.
Basic assignment statement
Multiple assignment statement
Augmented assignment statement
Chained assignment statement, unpacking assignment statement, swapping assignment statement.
Let’s see about each one in detail.
Basic Assignment Statement
The most frequently and commonly used is the basic assignment statement. In this type of assignment, we will assign the value to the variable directly. Following is the syntax.
Variable_name is the name of the variable.
value is the any datatype of input value to be assigned to the variable.
In this example, we are assigning a value to the variable using the basic assignment statement in the static manner.
In this example, we will use the dynamic inputting way to assign the value using the basic assignment statement.
Multiple Assignment statement
We can assign multiple values to multiple variables within a single line of code in python. Following is the syntax.
v1,v2,……,vn are the variable names.
val1,val2,……,valn are the values.
In this example, we will assign multiple values to the multiple variables using the multiple assignment statement.
By using the augmented assignment statement, we can combine the arithmetic or bitwise operations with the assignment. Following is the syntax.
variable is the variable name.
value is the input value.
+= is the assignment operator with the arithmetic operator.
In this example, we will use the augmented assignment statement to assign the values to the variable.
By using the chained assignment statement, we can assign a single value to the multiple variables within a single line. Following is the syntax -
v1,v2,v3 are the variable names.
value is the value to be assigned to the variables.
Here is the example to assign the single value to the multiple variables using the chain assignment statement.
We can assign the values given in a list or tuple can be assigned to multiple variables using the unpacking assignment statement. Following is the syntax -
val1,val2,val3 are the values.
In this example, we will assign the values grouped in the list to the multiple variables using the unpacking assignment statement.
In python, we can swap two values of the variables without using any temporary third variable with the help of assignment statement. Following is the syntax.
var1, var2 are the variables.
In the following example, we will assign the values two variables and swap the values with each other.

- Related Articles
- What is assignment statements with Integer types in compiler design?
- Give the different forms of silica in nature.
- A += B Assignment Riddle in Python
- Python – Priority key assignment in dictionary
- Python Program to Minimum Value Key Assignment
- What is vertical bar in Python bitwise assignment operator?
- Multiple Statements in Python
- Multi-Line Statements in Python
- Loop Control Statements in Python
- The import Statements in Python
- What are the different types of conditional statements supported by C#?
- Short Circuit Assignment in JavaScript
- Compound Assignment Operators in C++
- Compound assignment operators in C#
- Assignment operators in Dart Programming
Kickstart Your Career
Get certified by completing the course
- Python Tutorial
- Interview Questions
- Python Quiz
- Python Projects
- Practice Python
- Data Science With Python
- Python Web Dev
- DSA with Python
- Python OOPs
Assignment Operators in Python
The Python Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, and bitwise computations. The value the operator operates on is known as the Operand.
Assignment Operators are used to assign values to variables. This operator is used to assign the value of the right side of the expression to the left side operand.
Addition Assignment Operator
The Addition Assignment Operator is used to add the right-hand side operand with the left-hand side operand and then assigning the result to the left operand.
S ubtraction Assignment Operator
The Subtraction Assignment Operator is used to subtract the right-hand side operand from the left-hand side operand and then assigning the result to the left-hand side operand.
M ultiplication Assignment Operator
The Multiplication Assignment Operator is used to multiply the right-hand side operand with the left-hand side operand and then assigning the result to the left-hand side operand.
D ivision Assignment Operator
The Division Assignment Operator is used to divide the left-hand side operand with the right-hand side operand and then assigning the result to the left operand.
M odulus Assignment Operator
The Modulus Assignment Operator is used to take the modulus, that is, it first divides the operands and then takes the remainder and assigns it to the left operand.
F loor Division Assignment Operator
The Floor Division Assignment Operator is used to divide the left operand with the right operand and then assigs the result(floor value) to the left operand.
Exponentiation Assignment Operator
The Exponentiation Assignment Operator is used to calculate the exponent(raise power) value using operands and then assigning the result to the left operand.
Bitwise AND Assignment Operator
The Bitwise AND Assignment Operator is used to perform Bitwise AND operation on both operands and then assigning the result to the left operand.
Bitwise OR Assignment Operator
The Bitwise OR Assignment Operator is used to perform Bitwise OR operation on the operands and then assigning result to the left operand.
Bitwise XOR Assignment Operator
The Bitwise XOR Assignment Operator is used to perform Bitwise XOR operation on the operands and then assigning result to the left operand.
Bitwise Right Shift Assignment Operator
The Bitwise Right Shift Assignment Operator is used to perform Bitwise Right Shift Operation on the operands and then assign result to the left operand.
Bitwise Left Shift Assignment Operator
The Bitwise Left Shift Assignment Operator is used to perform Bitwise Left Shift Opertator on the operands and then assign result to the left operand.
Walrus Operator
The Walrus Operator in Python is a new assignment operator which is introduced in Python version 3.8 and higher. This operator is used to assign a value to a variable within an expression.
Example: In this code, we have a Python list of integers. We have used Python Walrus assignment operator within the Python while loop . The operator will solve the expression on the right-hand side and assign the value to the left-hand side operand ‘x’ and then execute the remaining code.
Assignment Operators in Python – FAQs
What are assignment operators in python.
Assignment operators in Python are used to assign values to variables. These operators can also perform additional operations during the assignment. The basic assignment operator is = , which simply assigns the value of the right-hand operand to the left-hand operand. Other common assignment operators include += , -= , *= , /= , %= , and more, which perform an operation on the variable and then assign the result back to the variable.
What is the := Operator in Python?
The := operator, introduced in Python 3.8, is known as the “walrus operator”. It is an assignment expression, which means that it assigns values to variables as part of a larger expression. Its main benefit is that it allows you to assign values to variables within expressions, including within conditions of loops and if statements, thereby reducing the need for additional lines of code. Here’s an example: # Example of using the walrus operator in a while loop while (n := int(input("Enter a number (0 to stop): "))) != 0: print(f"You entered: {n}") This loop continues to prompt the user for input and immediately uses that input in both the condition check and the loop body.
What is the Assignment Operator in Structure?
In programming languages that use structures (like C or C++), the assignment operator = is used to copy values from one structure variable to another. Each member of the structure is copied from the source structure to the destination structure. Python, however, does not have a built-in concept of ‘structures’ as in C or C++; instead, similar functionality is achieved through classes or dictionaries.
What is the Assignment Operator in Python Dictionary?
In Python dictionaries, the assignment operator = is used to assign a new key-value pair to the dictionary or update the value of an existing key. Here’s how you might use it: my_dict = {} # Create an empty dictionary my_dict['key1'] = 'value1' # Assign a new key-value pair my_dict['key1'] = 'updated value' # Update the value of an existing key print(my_dict) # Output: {'key1': 'updated value'}
What is += and -= in Python?
The += and -= operators in Python are compound assignment operators. += adds the right-hand operand to the left-hand operand and assigns the result to the left-hand operand. Conversely, -= subtracts the right-hand operand from the left-hand operand and assigns the result to the left-hand operand. Here are examples of both: # Example of using += a = 5 a += 3 # Equivalent to a = a + 3 print(a) # Output: 8 # Example of using -= b = 10 b -= 4 # Equivalent to b = b - 4 print(b) # Output: 6 These operators make code more concise and are commonly used in loops and iterative data processing.
Similar Reads
- Python Operators In Python programming, Operators in general are used to perform operations on values and variables. These are standard symbols used for logical and arithmetic operations. In this article, we will look into different types of Python operators. OPERATORS: These are the special symbols. Eg- + , * , /, 12 min read
- Precedence and Associativity of Operators in Python In Python, operators have different levels of precedence, which determine the order in which they are evaluated. When multiple operators are present in an expression, the ones with higher precedence are evaluated first. In the case of operators with the same precedence, their associativity comes int 4 min read
Python Arithmetic Operators
- Python Arithmetic Operators Python operators are fundamental for performing mathematical calculations. Arithmetic operators are symbols used to perform mathematical operations on numerical values. Arithmetic operators include addition (+), subtraction (-), multiplication (*), division (/), and modulus (%). Addition OperatorIn 4 min read
- Difference between / vs. // operator in Python In this article, we will see the difference between the / vs // operator in Python Python Division OperatorThe division operator '/ ' performs standard division, which can result in a floating-point number. However, if both the dividend and divisor are integers, Python will perform integer division 2 min read
- Python - Star or Asterisk operator ( * ) There are a many places you’ll see * and ** used in Python. Many Python Programmers even at the intermediate level are often puzzled when it comes to the asterisk ( * ) character in Python. After studying this article, you will have a solid understanding of the asterisk ( * ) operator in Python and 3 min read
- What does the Double Star operator mean in Python? Double Star or (**) is one of the Arithmetic Operator (Like +, -, *, **, /, //, %) in Python Language. It is also known as Power Operator. What is the Precedence of Arithmetic Operators? Arithmetic operators follow the same precedence rules as in mathematics, and they are: exponential is performed f 3 min read
- Division Operators in Python Division Operators allow you to divide two numbers and return a quotient, i.e., the first number or number at the left is divided by the second number or number at the right and returns the quotient. Division Operators in PythonThere are two types of division operators: Float divisionInteger divisio 6 min read
- Modulo operator (%) in Python When we see a '%' the first thing that comes to our mind is the "percent" but in computer language, it means modulo operation(%) which returns the remainder of dividing the left-hand operand by right-hand operand or in layman's terms it finds the remainder or signed remainder after the division of o 4 min read
Python Logical Operators
- Python Logical Operators Python logical operators are used to combine conditional statements, allowing you to perform operations based on multiple conditions. These Python operators, alongside arithmetic operators, are special symbols used to carry out computations on values and variables. In this article, we will discuss l 6 min read
- Python OR Operator Python OR Operator takes at least two boolean expressions and returns True if any one of the expressions is True. If all the expressions are False then it returns False. Flowchart of Python OR OperatorTruth Table for Python OR OperatorExpression 1Expression 2ResultTrueTrueTrueTrueFalseTrueFalseTrueT 4 min read
- Difference between 'and' and '&' in Python and is a Logical AND that returns True if both the operands are true whereas '&' is a bitwise operator in Python that acts on bits and performs bit-by-bit operations. Note: When an integer value is 0, it is considered as False otherwise True when used logically. and in PythonThe 'and' keyword in 6 min read
- not Operator in Python | Boolean Logic Python not keyword is a logical operator that is usually used to figure out the negation or opposite boolean value of the operand. The Keyword 'not' is a unary type operator which means that it takes only one operand for the logical operation and returns the complementary of the boolean value of the 5 min read
- Ternary Operator in Python The ternary operator in Python allows us to perform conditional checks and assign values or perform operations on a single line. It is also known as a conditional expression because it evaluates a condition and returns one value if the condition is True and another if it is False. Basic Example of T 5 min read
Python Bitwise Operators
- Python Bitwise Operators Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic and logical computations. The value the operator operates on is known as the Operand. Python bitwise operators are used to perform bitwise calculations on integers. The integers 6 min read

Python Assignment Operators
- Assignment Operators in Python The Python Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, and bitwise computations. The value the operator operates on is known as the Operand. Assignment Operators are used to assign values to variables. This opera 7 min read
- Walrus Operator in Python 3.8 The Walrus Operator is a new addition to Python 3.8 and higher. In this article, we're going to discuss the Walrus operator and explain it with an example. Introduction Walrus Operator allows you to assign a value to a variable within an expression. This can be useful when you need to use a value mu 2 min read
- Increment += and Decrement -= Assignment Operators in Python If you're familiar with Python, you would have known Increment and Decrement operators ( both pre and post) are not allowed in it. Python is designed to be consistent and readable. One common error by a novice programmer in languages with ++ and -- operators are mixing up the differences (both in pr 3 min read
- Merging and Updating Dictionary Operators in Python 3.9 Python 3.9 is still in development and scheduled to be released in October this year. On Feb 26, alpha 4 versions have been released by the development team. One of the latest features in Python 3.9 is the merge and update operators. There are various ways in which Dictionaries can be merged by the 3 min read
- New '=' Operator in Python3.8 f-string Python have introduced the new = operator in f-string for self documenting the strings in Python 3.8.2 version. Now with the help of this expression we can specify names in the string to get the exact value in the strings despite the position of the variable. Now f-string can be defined as f'{expr=} 1 min read
Python Relational Operators
- Comparison Operators in Python Python operators can be used with various data types, including numbers, strings, boolean and more. In Python, comparison operators are used to compare the values of two operands (elements being compared). When comparing strings, the comparison is based on the alphabetical order of their characters 4 min read
- Python NOT EQUAL operator In this article, we are going to see != (Not equal) operators. In Python, != is defined as not equal to operator. It returns True if operands on either side are not equal to each other, and returns False if they are equal. Python NOT EQUAL operators SyntaxThe Operator not equal in the Python descrip 3 min read
- Difference between == and is operator in Python In Python, == and is operators are both used for comparison but they serve different purposes. The == operator checks for equality of values which means it evaluates whether the values of two objects are the same. On the other hand, is operator checks for identity, meaning it determines whether two 4 min read
- Chaining comparison operators in Python Checking more than two conditions is very common in Programming Languages. Let's say we want to check the below condition: a < b < c The most common syntax to do it is as follows: if a < b and b < c : {...} In Python, there is a better way to write this using the Comparison operator Chai 5 min read
- Python Membership and Identity Operators There is a large set of Python operators that can be used on different datatypes. Sometimes we need to know if a value belongs to a particular set. This can be done by using the Membership and Identity Operators. In this article, we will learn about Python Membership and Identity Operators. Table of 5 min read
- Difference between != and is not operator in Python In this article, we are going to see != (Not equal) operators. In Python != is defined as not equal to operator. It returns True if operands on either side are not equal to each other, and returns False if they are equal. Whereas is not operator checks whether id() of two objects is same or not. If 3 min read
- Python-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python assignment operators.
Assignment operators are used to assign values to variables:
Related Pages

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
The Assignment Statement
Assignment is fundamental to Python; it is how the objects created by an expression are preserved. We'll look at the basic assignment statement, plus the augmented assignment statement. Later, in Multiple Assignment Statement , we'll look at multiple assignment.
Basic Assignment
We create and change variables primarily with the assignment statement. This statement provides an expression and a variable name which will be used to label the value of the expression.
variable = expression
Here's a short script that contains some examples of assignment statements.
Example 6.1. example3.py
We have an object, the number 150 , which we assign to the variable shares . We have an expression 3+5.0/8.0 , which creates a floating-point number, which we save in the variable price . We have another expression, shares * price , which creates a floating-point number; we save this in value so that we can print it. This script created three new variables.
Since this file is new, we'll need to do the chmod +x example3.py once, after we create this file. Then, when we run this progam, we see the following.
Augmented Assignment
Any of the usual arithmetic operations can be combined with assignment to create an augmented assignment statement.
For example, look at this augmented assignment statement:
This statement is a shorthand that means the same thing as the following:
Here's a larger example
Example 6.2. portfolio.py
First, we'll do the chmod +x portfolio.py on this file. Then, when we run this progam, we see the following.
The other basic math operations can be used similarly, although the purpose gets obscure for some operations. These include -=, *=, /=, %=, &=, ^=, |=, <<= and >>=.
Here's a lengthy example. This is an extension of Craps Odds in the section called “Numeric Types and Expressions” .
In craps, the first roll of the dice is called the “ come out roll ”. This roll can be won immediately if one rolls 7 or 11. It can be lost immediately if one roll 2, 3 or 12. The remaining numbers establish a point and the game continues.
Example 6.3. craps.py
There's a 22.2% chance of winning, and a 11.1% chance of losing. What's the chance of establishing a point? One way is to figure that it's what's left after winning or loosing. The total of all probabilities always add to 1. Subtract the odds of winning and the odds of losing and what's left is the odds of setting a point.
Here's another way to figure the odds of rolling 4, 5, 6, 8, 9 or 10.
By the way, you can add the statement print win + lose + point to confirm that these odds all add to 1. This means that we have defined all possible outcomes for the come out roll in craps.
Tracing Execution
We can trace the execution of a program by simply following the changes of value of all the variables in the program. Here's an example for Example 6.3, “craps.py”
- win: 0 , 0.16 , 0.22
- list: 0 , 0.027 , 0.083 , 0.111
- point: 1 , 0.77 , 0.66
As with many things Python, there is some additional subtlety to this, but we'll cover those topics later. For example, multiple-assignment statement is something we'll look into in more deeply in Chapter 13, Tuples .

- Contributors
Basic Statements in Python
Table of contents, what is a statement in python, statement set, multi-line statements, simple statements, expression statements, the assert statement, the try statement.
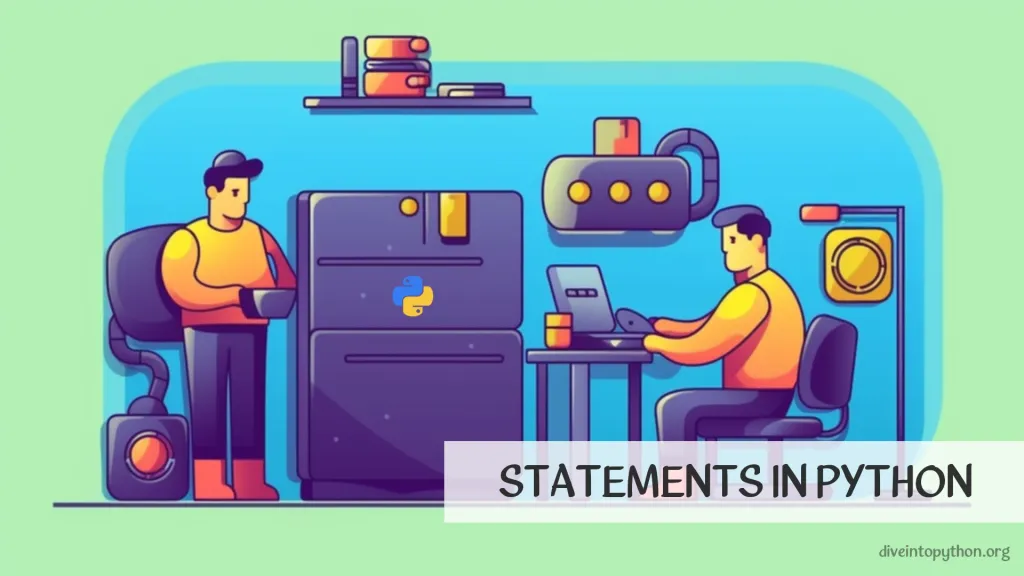
In Python, statements are instructions or commands that you write to perform specific actions or tasks. They are the building blocks of a Python program.
A statement is a line of code that performs a specific action. It is the smallest unit of code that can be executed by the Python interpreter.
Assignment Statement
In this example, the value 10 is assigned to the variable x using the assignment statement.
Conditional Statement
In this example, the if-else statement is used to check the value of x and print a corresponding message.
By using statements, programmers can instruct the computer to perform a variety of tasks, from simple arithmetic operations to complex decision-making processes. Proper use of statements is crucial to writing efficient and effective Python code.
Here's a table summarizing various types of statements in Python:
Please note that this table provides a brief overview of each statement type, and there may be additional details and variations for each statement.
Multi-line statements are a convenient way to write long code in Python without making it cluttered. They allow you to write several lines of code as a single statement, making it easier for developers to read and understand the code. Here are two examples of multi-line statements in Python:
- Using backslash:
- Using parentheses:
Simple statements are the smallest unit of execution in Python programming language and they do not contain any logical or conditional expressions. They are usually composed of a single line of code and can perform basic operations such as assigning values to variables , printing out values, or calling functions .
Examples of simple statements in Python:
Simple statements are essential to programming in Python and are often used in combination with more complex statements to create robust programs and applications.
Expression statements in Python are lines of code that evaluate and produce a value. They are used to assign values to variables, call functions, and perform other operations that produce a result.
In this example, we assign the value 5 to the variable x , then add 3 to x and assign the result ( 8 ) to the variable y . Finally, we print the value of y .
In this example, we define a function square that takes one argument ( x ) and returns its square. We then call the function with the argument 5 and assign the result ( 25 ) to the variable result . Finally, we print the value of result .
Overall, expression statements are an essential part of Python programming and allow for the execution of mathematical and computational operations.
The assert statement in Python is used to test conditions and trigger an error if the condition is not met. It is often used for debugging and testing purposes.
Where condition is the expression that is tested, and message is the optional error message that is displayed when the condition is not met.
In this example, the assert statement tests whether x is equal to 5 . If the condition is met, the statement has no effect. If the condition is not met, an error will be raised with the message x should be 5 .
In this example, the assert statement tests whether y is not equal to 0 before performing the division. If the condition is met, the division proceeds as normal. If the condition is not met, an error will be raised with the message Cannot divide by zero .
Overall, assert statements are a useful tool in Python for debugging and testing, as they can help catch errors early on. They are also easily disabled in production code to avoid any unnecessary overhead.
The try statement in Python is used to catch exceptions that may occur during the execution of a block of code. It ensures that even when an error occurs, the code does not stop running.
Examples of Error Processing
Dive deep into the topic.
- Match Statements
- Operators in Python Statements
- The IF Statement
Contribute with us!
Do not hesitate to contribute to Python tutorials on GitHub: create a fork, update content and issue a pull request.
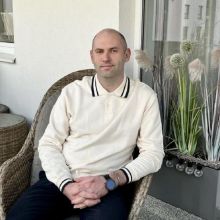
- Skip to main content
- Skip to primary sidebar
- Skip to secondary sidebar

Python Variables and Assignment Statements
Variables are storage locations that have a name. Said another way variables are name value pairs. You can assign values to a variable and recall those values by the variable name. To assign a value to a variable use the ‘=’ sign. The format is,
Here we have an example where the value of Apple is assigned to the variable called fruit.
You can change the value of the variable by reassigning it. Below you can see how to set the value of the fruit variable to the value of orange.
Note that there is nothing significant about the variable name fruit. We could have easily used almost any other variable name that you can think of. When choosing a variable name pick something that represents the data of the variable will hold.
You may know what a variable name X represents today, but if you come back to the code a few months from now you may not. However, if you encounter a variable name fruit chances are you can guess what type of data it will hold.
Variable names are case sensitive. A variable name fruit that begins with a capital F and another variable name fruit that is in all lowercase are two different variables. By convention variables are in all lowercase letter but it’s not a requirement.
Variable names must start with the letter. They can contain numbers but variable names cannot start with a number.
You can also use the underscore character in variable names. You cannot use +, – and some other various symbols in variable names. Whenever you get the urge to use a hyphen just use an underscore (_) instead.
Here are some examples of valid variable names.
The first example is in all lowercase letters and it contains a number. However, it doesn’t start with a number so it is a valid variable name.
The second line first_five_letters is in all lowercase and it includes underscores which are valid. However, you can’t use dashes so first-five-letters would not be a valid variable name.
The third variable is firstFiveLetters and it’s in mixed case some lowercase letters and some uppercase letters. Again this is perfectly fine, but just note that this variable would be a completely distinct from all lowercase variable name firstfiveletters for example.
In the Python interactive shell, you can run arithmetic operations without declaring it just as shown below.
In the vast majority of applications that you create and even in interactive mode you’re frequently going to want to store values for later use in your programs. The way that you do that is with variables just like in algebra. For example I can go ahead and create a variable with a simple statement like the one below.
The name of the variable that I have created is x and the value that I have given to x is the integer value 6.
In python anytime you see a whole number like 6 or 25 or 37, that is going to represent an integer value. Whenever you see a number that has a decimal point in it that’s going to represent a floating point value. And python just knows that as part of the languages definition.
Unlike up above when I execute this snippet there is no output for an assignment statement, so this is actually a statement in python that gives the value 6 to the variable x.
Some place in memory the value 6 is stored and we can now refer to that memory location as x and get the value at that location.
Python is a dynamically typed language so it figures out the type of this variable, not based on how you define it but based on what you assign to it.
So you give it the integer value 6 and python knows that at this moment in time x is an integer with the value 6. But because python is dynamically typed we can actually change that at a later time. We’ll learn that to you as we proceed through this tutorial.
So this is an assignment statement and similarly we could create another variable, we’ll call it y and let’s give it the value 3 here.
Now we have a couple of variables that we can use in the subsequent snippets of code.
Variables in algebra can be used in larger expressions. For example, we can do things like,
which is going to look up the value of x, look up the value of y, and then calculate the result. As we have declared above that x is 6, y is 3, so this is really 6+3. And when I press enter you can see that the Python environment immediately figures that out and displays the result for me.
The great thing about working in python interactive mode in this manner is that you can quickly see your results for every single statement or expression that you write. This enables you to really experiment with the language and learn the language very quickly.
Now, of course when we do an expression like x plus y we might want to store the result for later use. For that let’s go ahead and create a total variable and we’ll give it the value of x plus y.
so total gets us assigned the value of the expression x plus y, whenever you have an assignment expression or an assignment statement like this, whatever’s on the right side of the assignment is going to be evaluated first, and then the result will be stored into the variable on the left side of the assignment.
The type of the variable total will be determined by the type of evaluating the expression we have x which is the integer 6, we have y which is the integer 3. So when you add two integers you get an integer result and thus total also will be an integer. And at this point if I hit enter we see no output.
But in the python interactive session, you can always evaluate a variable to see what its current value is. So I can go ahead and type total and see that indeed total contains the value 9.
Variables x, y and total and even the numbers 25, 37, 6 and 3, all of these things have types associated with them. And it turns out that there is a bunch of so called built in functions in the python language that you can use. One of which is a function named ‘type’, which you can give it a value or a variable name and it will tell you the type of that value or variable.
If we want to determine what the type of the variable x is, we can just go ahead and type
And when I press enter here you’ll notice that it displays the type int, which is integer in the python language.
If I go ahead and type something like 15.3 inside the parentheses of the type function and press enter we can see that the type of that value is a float.
So at any point if you have a variable and you’re unsure of what its type is, and you’re working in a python interactive mode, you can simply pass that variables name to the type function and in turn it will give you back the corresponding type name.
I mentioned that type is a built in function. And also in order to know about all of the different built in functions that are available to you as part of the python language, go to https://docs.python.org/3/library/functions.html.
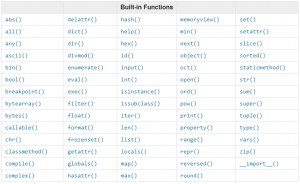
As you can see that there’s quite a number of built in functions available. And in python they tend to take the approach of providing as much as capability as possible to you so that you can focus on the larger task at hand.
Related posts:
- Arithmetic Operators in Python
You must be logged in to post a comment.
- C++ Language
- Python Hindi Tutorial
- Python Language
- Machine Learning
- Java Language
- C++ Programs
- Python Programs
- Java Programs
- mcq questions
- Privacy Policy
- DMCA policy
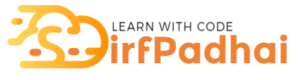
What are Assignment Statements in Python | Python assignment statement examples
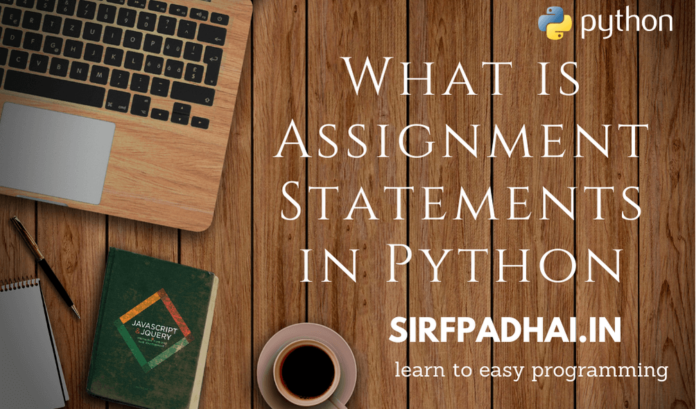
Table of Contents
What are Assignment Statements in Python
Assignments.
The assignment is the most basic statement in Python, assigning data and an object type to a variable name. You have already seen a number of different examples of this when you learned about the different Python object types. Unlike C, like Perl (in non-strict mode), you do not need to predeclare Python variables before you assign them a value. However, variables must exist when used within an expression or other form of a statement.
There are four basic methods of assignments: basic assignments, and tuple assignments. list assignment, and multiple-target assignment.
Basic Assignments
The basic assignment is the one you have seen most examples of in this chapter. A basic assignment creates a new object using the correct type according to the value assigned to the object, and then the name of the new object, as in the following examples:
Tuple and List Assignments
You can extract the individual elements from a tuple or list using a different operator of assignment. For tuples, you can just specify the list of variables to assign to, each separated by a comma:
What you’re in fact doing in the preceding statements is creating a new tuple on the left-hand side of the assignment operator. However, the tuple with the variable names is anonymous and the information about the entire tuple is not recorded. Because you don’t have to use parentheses to indicate a tuple, the assignment looks more natural, although you could have written them as:
What you end up with in each case is a new variable pointing to the information on the right-hand side. Note as well that you can also use slices and indexes to extract multiple elements from a tuple. For lists, you must create a new, anonymous list in the same way that you create an anonymous tuple:
The tuple and list assignments have another trick up their sleeve. Because names are merely pointers, you can “swap” two object/name combinations by performing a tuple or list assignment as follows:
You can see this better in the following interactive session:
Multiple-Target Assignments
You can create a single object with multiple pointers using the multiple-target assignment statement:
In the preceding statement, you created a single-string object with two different pointers called group and title. Remember that because the two pointers point at the same object, modifying the object contents using one name also modifies the information available via the other name. This is effectively the same as:
Unlike other languages, the default method for communicating with the user is via a statement rather than via a function. The statement has the same basic name, print, but is embedded into the interpreter. Unlike similar print functions, however, the Python print statement actually outputs string representations of the supplied objects to the standard output of the interpreter. The destination is the same as the C stdout or Perl STDOUT file handles, and cannot normally be pointed elsewhere.
Actually, you can redirect the standard output by modifying the object used to paint to the standard output device. It should be no surprise that Python uses objects to communicate with the outside world. The following code demonstrates this point:
This works because instead of pointing the standard output to its original location, you copy the object data for the new fp object instead. The stdout object, which is used by the print function, now sends all of the output to the file somefile. txt .
When printing, the statement follows these basic rules:
•Objects and constants separated by commas are separated by spaces when printed. Use string concatenation or the % formatting operator to avoid this.
•A line feed is appended to every output line. To avoid this, append a comma to the end of the line.
For example, the following script, when executed, displays the different forms supported:
The preceding script produces the following output:
Note how in the last line, print displays the complex object in the same format as str and the “operator.
The ability to print out the contents of an object in this way is extremely useful, both as a programming tool and for debugging. For example, you can store the configuration parameters for an application in one or more variables. When you want to save the information, just use print or the str function to write out the statements. To get the information back, all you need do is import the file contents in the same way that you would with any other module.
RELATED ARTICLES MORE FROM AUTHOR
Python numpy tutorial sirfpadhai, 100 days of python code pdf | python cheat sheet, python text manipulation(splitting)|python text manipulation tutorial.
[…] Assignments […]
LEAVE A REPLY Cancel reply
Save my name, email, and website in this browser for the next time I comment.
Sirfpadhai.in
Here you are provided with tutorials related to Computer Science, Web Development in Hindi with information related to Programming Language like C, C++, Java, Python. You can practice objective questions here. Thanks for visiting our website.
Contact us: [email protected]
cloud computing framework model based on the SOA.
What is soa(service-oriented architecture).

IMAGES
COMMENTS
May 10, 2020 · There are some important properties of assignment in Python :-Assignment creates object references instead of copying the objects. Python creates a variable name the first time when they are assigned a value. Names must be assigned before being referenced. There are some operations that perform assignments implicitly. Assignment statement forms ...
The as keyword in with statements, except clauses, and import statements is another example of an implicit assignment in Python. This time, the assignment isn’t completely implicit because the as keyword provides an explicit way to define the target variable.
Oct 20, 2023 · Assignment statement in python is the statement used to assign the value to the specified variable. The value assigned to the variable can be of any data type supported by python programming language such as integer, string, float Boolean, list, tuple, dictionary, set etc. Types of assignment statements
Aug 30, 2021 · A statement is an instruction that a Python interpreter can execute. So, in simple words, we can say anything written in Python is a statement. Python statement ends with the token NEWLINE character. It means each line in a Python script is a statement. For example, a = 10 is an assignment statement. where a is a variable name and
Dec 4, 2024 · The Walrus Operator in Python is a new assignment operator which is introduced in Python version 3.8 and higher. This operator is used to assign a value to a variable within an expression. Syntax: a := expression. Example: In this code, we have a Python list of integers. We have used Python Walrus assignment operator within the Python while loop.
Python Examples Python Examples ... Python Assignment Operators. Assignment operators are used to assign values to variables: Operator Example Same As Try it = x = 5 ...
Any of the usual arithmetic operations can be combined with assignment to create an augmented assignment statement. For example, look at this augmented assignment statement: a += v This statement is a shorthand that means the same thing as the following: a = a + v Here's a larger example
May 3, 2024 · Assignment Statement x = 10 In this example, the value 10 is assigned to the variable x using the assignment statement. Conditional Statement x = 3 if x < 5: print("x is less than 5") else: print("x is greater than or equal to 5") In this example, the if-else statement is used to check the value of x and print a corresponding message.
For example I can go ahead and create a variable with a simple statement like the one below. x = 6 Code language: Python (python) The name of the variable that I have created is x and the value that I have given to x is the integer value 6. In python anytime you see a whole number like 6 or 25 or 37, that is going to represent an integer value.
Jul 1, 2024 · The assignment is the most basic statement in Python, assigning data and an object type to a variable name. You have already seen a number of different examples of this when you learned about the different Python object types. Unlike C, like Perl (in non-strict mode), you do not need to predeclare Python variables before you assign them a value.