- Language Reference

Assignment Operators
The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". Don't. It really means that the left operand gets set to the value of the expression on the right (that is, "gets set to").
The value of an assignment expression is the value assigned. That is, the value of " $a = 3 " is 3. This allows you to do some tricky things: <?php $a = ( $b = 4 ) + 5 ; // $a is equal to 9 now, and $b has been set to 4. ?>
In addition to the basic assignment operator, there are "combined operators" for all of the binary arithmetic , array union and string operators that allow you to use a value in an expression and then set its value to the result of that expression. For example: <?php $a = 3 ; $a += 5 ; // sets $a to 8, as if we had said: $a = $a + 5; $b = "Hello " ; $b .= "There!" ; // sets $b to "Hello There!", just like $b = $b . "There!"; ?>
Note that the assignment copies the original variable to the new one (assignment by value), so changes to one will not affect the other. This may also have relevance if you need to copy something like a large array inside a tight loop.
An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference. Objects may be explicitly copied via the clone keyword.
Assignment by Reference
Assignment by reference is also supported, using the " $var = &$othervar; " syntax. Assignment by reference means that both variables end up pointing at the same data, and nothing is copied anywhere.
Example #1 Assigning by reference
The new operator returns a reference automatically, as such assigning the result of new by reference is an error.
The above example will output:
More information on references and their potential uses can be found in the References Explained section of the manual.
Arithmetic Assignment Operators
Bitwise assignment operators, other assignment operators.
- arithmetic operators
- bitwise operators
- null coalescing operator
Found A Problem?
User contributed notes 4 notes.

Home » PHP Tutorial » PHP Assignment Operators
PHP Assignment Operators
Summary : in this tutorial, you will learn about the most commonly used PHP assignment operators.
Introduction to the PHP assignment operator
PHP uses the = to represent the assignment operator. The following shows the syntax of the assignment operator:
On the left side of the assignment operator ( = ) is a variable to which you want to assign a value. And on the right side of the assignment operator ( = ) is a value or an expression.
When evaluating the assignment operator ( = ), PHP evaluates the expression on the right side first and assigns the result to the variable on the left side. For example:
In this example, we assigned 10 to $x, 20 to $y, and the sum of $x and $y to $total.
The assignment expression returns a value assigned, which is the result of the expression in this case:
It means that you can use multiple assignment operators in a single statement like this:
In this case, PHP evaluates the right-most expression first:
The variable $y is 20 .
The assignment expression $y = 20 returns 20 so PHP assigns 20 to $x . After the assignments, both $x and $y equal 20.
Arithmetic assignment operators
Sometimes, you want to increase a variable by a specific value. For example:
How it works.
- First, $counter is set to 1 .
- Then, increase the $counter by 1 and assign the result to the $counter .
After the assignments, the value of $counter is 2 .
PHP provides the arithmetic assignment operator += that can do the same but with a shorter code. For example:
The expression $counter += 1 is equivalent to the expression $counter = $counter + 1 .
Besides the += operator, PHP provides other arithmetic assignment operators. The following table illustrates all the arithmetic assignment operators:
Concatenation assignment operator
PHP uses the concatenation operator (.) to concatenate two strings. For example:
By using the concatenation assignment operator you can concatenate two strings and assigns the result string to a variable. For example:
- Use PHP assignment operator ( = ) to assign a value to a variable. The assignment expression returns the value assigned.
- Use arithmetic assignment operators to carry arithmetic operations and assign at the same time.
- Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
PHP Tutorial
Php advanced, mysql database, php examples, php reference, php operators.
Operators are used to perform operations on variables and values.
PHP divides the operators in the following groups:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Increment/Decrement operators
- Logical operators
- String operators
- Array operators
- Conditional assignment operators
PHP Arithmetic Operators
The PHP arithmetic operators are used with numeric values to perform common arithmetical operations, such as addition, subtraction, multiplication etc.
PHP Assignment Operators
The PHP assignment operators are used with numeric values to write a value to a variable.
The basic assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right.
Advertisement
PHP Comparison Operators
The PHP comparison operators are used to compare two values (number or string):
PHP Increment / Decrement Operators
The PHP increment operators are used to increment a variable's value.
The PHP decrement operators are used to decrement a variable's value.
PHP Logical Operators
The PHP logical operators are used to combine conditional statements.
PHP String Operators
PHP has two operators that are specially designed for strings.
PHP Array Operators
The PHP array operators are used to compare arrays.
PHP Conditional Assignment Operators
The PHP conditional assignment operators are used to set a value depending on conditions:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- PHP Tutorial
- PHP Exercises
- PHP Calendar
- PHP Filesystem
- PHP Programs
- PHP Array Programs
- PHP String Programs
- PHP Interview Questions
- PHP IntlChar
- PHP Image Processing
- PHP Formatter
- Web Technology
PHP Operators
In this article, we will see how to use the operators in PHP, & various available operators along with understanding their implementation through the examples.
Operators are used to performing operations on some values. In other words, we can describe operators as something that takes some values, performs some operation on them, and gives a result. From example, “1 + 2 = 3” in this expression ‘+’ is an operator. It takes two values 1 and 2, performs an addition operation on them to give 3.
Just like any other programming language, PHP also supports various types of operations like arithmetic operations(addition, subtraction, etc), logical operations(AND, OR etc), Increment/Decrement Operations, etc. Thus, PHP provides us with many operators to perform such operations on various operands or variables, or values. These operators are nothing but symbols needed to perform operations of various types. Given below are the various groups of operators:
- Arithmetic Operators
- Logical or Relational Operators
- Comparison Operators
- Conditional or Ternary Operators
- Assignment Operators
- Spaceship Operators (Introduced in PHP 7)
- Array Operators
- Increment/Decrement Operators
- String Operators
Let us now learn about each of these operators in detail.
Arithmetic Operators:
The arithmetic operators are used to perform simple mathematical operations like addition, subtraction, multiplication, etc. Below is the list of arithmetic operators along with their syntax and operations in PHP.
Note : The exponentiation has been introduced in PHP 5.6.
Example : This example explains the arithmetic operator in PHP.
Logical or Relational Operators:
These are basically used to operate with conditional statements and expressions. Conditional statements are based on conditions. Also, a condition can either be met or cannot be met so the result of a conditional statement can either be true or false. Here are the logical operators along with their syntax and operations in PHP.
Example : This example describes the logical & relational operator in PHP.
Comparison Operators: These operators are used to compare two elements and outputs the result in boolean form. Here are the comparison operators along with their syntax and operations in PHP.
Example : This example describes the comparison operator in PHP.
Conditional or Ternary Operators :
These operators are used to compare two values and take either of the results simultaneously, depending on whether the outcome is TRUE or FALSE. These are also used as a shorthand notation for if…else statement that we will read in the article on decision making.
Here, the condition will either evaluate as true or false. If the condition evaluates to True, then value1 will be assigned to the variable $var otherwise value2 will be assigned to it.
Example : This example describes the Conditional or Ternary operators in PHP.
Assignment Operators: These operators are used to assign values to different variables, with or without mid-operations. Here are the assignment operators along with their syntax and operations, that PHP provides for the operations.
Example : This example describes the assignment operator in PHP.
Array Operators: These operators are used in the case of arrays. Here are the array operators along with their syntax and operations, that PHP provides for the array operation.
Example : This example describes the array operation in PHP.
Increment/Decrement Operators: These are called the unary operators as they work on single operands. These are used to increment or decrement values.
Example : This example describes the Increment/Decrement operators in PHP.
String Operators: This operator is used for the concatenation of 2 or more strings using the concatenation operator (‘.’). We can also use the concatenating assignment operator (‘.=’) to append the argument on the right side to the argument on the left side.
Example : This example describes the string operator in PHP.
Spaceship Operators :
PHP 7 has introduced a new kind of operator called spaceship operator. The spaceship operator or combined comparison operator is denoted by “<=>“. These operators are used to compare values but instead of returning the boolean results, it returns integer values. If both the operands are equal, it returns 0. If the right operand is greater, it returns -1. If the left operand is greater, it returns 1. The following table shows how it works in detail:
Example : This example illustrates the use of the spaceship operator in PHP.
Similar Reads
- PHP Tutorial PHP (Hypertext Preprocessor) is a versatile and widely used server-side scripting language for creating dynamic and interactive web applications. This PHP tutorial will give you an in-depth understanding of the PHP scripting language. Whether you are a beginner or a professional PHP developer this f 6 min read
- PHP Introduction The term PHP is an acronym for - Hypertext Preprocessor. PHP is a server-side scripting language designed specifically for web development. It is an open-source which means it is free to download and use. It is very simple to learn and use. The file extension of PHP is ".php". What is PHP?PHP is a s 8 min read
- How to install PHP in Windows 10? PHP is a general-purpose scripting language geared towards web development. It is an open-source software (OSS), which is free to download and use. PHP stands for "Hypertext Preprocessor". PHP files are saved with an extension called .php. It supports many databases MySQL, Oracle, etc. Installation 2 min read
- How to Install PHP on Linux? PHP is a popular server-side scripting language that is especially used in web development. If you're working on a Linux environment, whether it's a personal development setup or a production server, you will likely need PHP installed. In this article, we will see the step-by-step guide to install P 2 min read
- PHP Syntax PHP, a powerful server-side scripting language used in web development. It’s simplicity and ease of use makes it an ideal choice for beginners and experienced developers. This article provides an overview of PHP syntax. PHP scripts can be written anywhere in the document within PHP tags along with n 4 min read
- How to write comments in PHP ? Comments are non-executable lines of text in the code that are ignored by the PHP interpreter. Comments are an essential part of any programming language. It help developers to understand the code, provide explanations, and make the codebase more maintainable. Types of Comments in PHPPHP supports tw 1 min read
- PHP Variables PHP Variables are one of the most fundamental concepts in programming. It is used to store data that can be accessed and manipulated within your code. Variables in PHP are easy to use, dynamically typed (meaning that you do not need to declare their type explicitly), and essential for creating dynam 5 min read
- PHP echo and print PHP echo and print are two most language constructs used for output data on the screen. They are not functions but constructs, meaning they do not require parentheses (though parentheses can be used with print). Both are widely used for displaying strings, variables, and HTML content in PHP scripts. 5 min read
- PHP Data Types PHP data types are a fundamental concept to defines how variables store and manipulate data. PHP is a loosely typed language, which means variables do not need to be declared with a specific data type. PHP allows eight different types of data types. All of them are discussed below. Table of Content 5 min read
- PHP Strings PHP Strings are one of the most fundamental data types. It is used to handle text data, manipulate user inputs, generate dynamic content, and much more. PHP provides a wide array of functions and techniques for working with strings, making it easy to manipulate and display text. A string is a sequen 7 min read
- PHP Arrays Arrays in PHP are a type of data structure that allows us to store multiple elements of similar or different data types under a single variable thereby saving us the effort of creating a different variable for every data. The arrays are helpful to create a list of elements of similar types, which ca 6 min read
- Associative Arrays in PHP Associative arrays are used to store key value pairs. For example, to store the marks of different subject of a student in an array, a numerically indexed array would not be the best choice. Instead, we could use the respective subject's names as the keys in our associative array, and the value woul 3 min read
- Multidimensional arrays in PHP Multi-dimensional arrays in PHP are arrays that store other arrays as their elements. Each dimension adds complexity, requiring multiple indices to access elements. Common forms include two-dimensional arrays (like tables) and three-dimensional arrays, useful for organizing complex, structured data. 5 min read
- Sorting Arrays in PHP Sorting arrays is one of the most common operation in programming, and PHP provides a several functions to handle array sorting. Sorting arrays in PHP can be done by values or keys, in ascending or descending order. PHP also allows you to create custom sorting functions. Table of Content Sort Array 4 min read
PHP Constants
- PHP Constants Constants in PHP are identifiers or names that can be assigned any fixed values and cannot change during the execution of a program. They remain constant throughout the program and cannot be changed during the execution. Once a constant is defined, it cannot be undefined or redefined. By convension, 2 min read
- PHP Constant Class The const keyword is used to declare a class constant. A constant is unchangeable once it is declared. The constant class declared inside the class definition. The class constant is case-sensitive. However, it is recommended to name the constants in all uppercase letters. Constant differ from normal 2 min read
- PHP Defining Constants In a production-level code, it is very important to keep the information as either variables or constants rather than using them explicitly. A PHP constant is nothing but an identifier for a simple value that tends not to change over time(such as the domain name of a website eg. www.geeksforgeeks.or 2 min read
- PHP | Magic Constants Magic constants: Magic constants are the predefined constants in PHP which is used on the basis of their use. These constants are created by various extensions. There are nine magic constant in the PHP and all of the constant resolved at the compile-time, not like the regular constant which is resol 3 min read
- PHP Operators In this article, we will see how to use the operators in PHP, & various available operators along with understanding their implementation through the examples. Operators are used to performing operations on some values. In other words, we can describe operators as something that takes some value 10 min read
- PHP | Bitwise Operators The Bitwise operators is used to perform bit-level operations on the operands. The operators are first converted to bit-level and then calculation is performed on the operands. The mathematical operations such as addition , subtraction , multiplication etc. can be performed at bit-level for faster p 5 min read
- PHP | Ternary Operator If-else and Switch cases are used to evaluate conditions and decide the flow of a program. The ternary operator is a shortcut operator used for shortening the conditional statements. ternary operator: The ternary operator (?:) is a conditional operator used to perform a simple comparison or check on 3 min read
PHP Control Statements
- PHP | Decision Making PHP allows us to perform actions based on some type of conditions that may be logical or comparative. Based on the result of these conditions i.e., either TRUE or FALSE, an action would be performed as asked by the user. It's just like a two- way path. If you want something then go this way or else 4 min read
- PHP switch Statement The switch statement is similar to the series of if-else statements. The switch statement performs in various cases i.e. it has various cases to which it matches the condition and appropriately executes a particular case block. It first evaluates an expression and then compares it with the values of 2 min read
- PHP break (Single and Nested Loops) In PHP break is used to immediately terminate the loop and the program control resumes at the next statement following the loop. Method 1: Given an array the task is to run a loop and display all the values in array and terminate the loop when encounter 5. Examples: Input : array1 = array( 1, 2, 3, 2 min read
- PHP continue Statement The continue statement is used within a loop structure to skip the loop iteration and continue execution at the beginning of condition execution. It is mainly used to skip the current iteration and check for the next condition. The continue accepts an optional numeric value that tells how many loops 1 min read
- PHP Loops Like other programming languages, PHP Loops are used to repeat a block of code multiple times based on a given condition. PHP provides several types of loops to handle different scenarios, including while loops, for loops, do...while loops, and foreach loops. In this article, we will explore the dif 3 min read
- PHP while Loop The while loop is the simple loop that executes nested statements repeatedly while the expression value is true. The expression is checked every time at the beginning of the loop, and if the expression evaluates to true then the loop is executed otherwise loop is terminated. Flowchart of While Loop: 1 min read
- PHP do-while Loop The do-while loop is very similar to the while loop, the only difference is that the do-while loop checks the expression (condition) at the end of each iteration. In a do-while loop, the loop is executed at least once when the given expression is "false". The first iteration of the loop is executed 1 min read
- PHP for Loop The for loop is the most complex loop in PHP that is used when the user knows how many times the block needs to be executed. The for loop contains the initialization expression, test condition, and update expression (expression for increment or decrement). Flowchart of for Loop: Syntax: for (initial 2 min read
- PHP foreach Loop The foreach loop in PHP is a powerful and convenient way to iterate over arrays and objects. The foreach loop though iterates over an array of elements, the execution is simplified and finishes the loop in less time comparatively. In this article, we will explore the foreach loop in detail, includin 3 min read
PHP Functions
- PHP | Functions A function is a block of code written in a program to perform some specific task. We can relate functions in programs to employees in a office in real life for a better understanding of how functions work. Suppose the boss wants his employee to calculate the annual budget. So how will this process c 6 min read
- PHP Arrow Functions Arrow functions, also known as "short closures", is a new feature introduced in PHP 7.4 that provides a more concise syntax for defining anonymous functions. Arrow functions allow you to define a function in a single line of code, making your code more readable and easier to maintain. Syntax: $fn = 1 min read
- Anonymous recursive function in PHP Anonymous recursive function is a type of recursion in which function does not explicitly call another function by name. This can be done either comprehensively, by using a higher order function passing in a function as an argument and calling that function. It can be done implicitly, via reflection 2 min read
PHP Advanced
- PHP | Superglobals We already have discussed about variables and global variables in PHP in the post PHP | Variables and Data Types. In this article, we will learn about superglobals in PHP. These are specially-defined array variables in PHP that make it easy for you to get information about a request or its context. 5 min read
- HTTP GET and POST Methods in PHP In this article, we will know what HTTP GET and POST methods are in PHP, how to implement these HTTP methods & their usage, by understanding them through the examples. HTTP: The Hypertext Transfer Protocol (HTTP) is designed to enable communications between clients and servers. HTTP works as a r 4 min read
- PHP | Regular Expressions Regular expressions commonly known as a regex (regexes) are a sequence of characters describing a special search pattern in the form of text string. They are basically used in programming world algorithms for matching some loosely defined patterns to achieve some relevant tasks. Some times regexes a 11 min read
- PHP Form Processing In this article, we will discuss how to process form in PHP. HTML forms are used to send the user information to the server and returns the result back to the browser. For example, if you want to get the details of visitors to your website, and send them good thoughts, you can collect the user infor 5 min read
- PHP Date and Time In this article, we will see how to get the date & time using the date() & time() function in PHP, we will also see the various formatting options available with these functions & understand their implementation through the examples. Date and time are some of the most frequently used ope 4 min read
- Describe PHP Include and Require In this article, we will see what include() & the require() functions is, also will know how these functions affect the execution of the code, their differences & usage in PHP, along with understanding their implementation through the examples. As we know PHP allows us to create various func 3 min read
- PHP File Handling File handling in PHP is used to you to create, open, read, write, delete, and manipulate files on a server. It is used when you need to store data persistently or handle files uploaded by users. PHP provides several built-in functions to make file handling easy and secure. Table of Content Common Fi 3 min read
- PHP | Uploading File Have you ever wondered how websites build their system of file uploading in PHP? Here we will come to know about the file uploading process. A question which you can come up with - 'Are we able to upload any kind of file with this system?'. The answer is yes, we can upload files with different types 3 min read
- PHP Cookies A cookie in PHP is a small file with a maximum size of 4KB that the web server stores on the client computer. They are typically used to keep track of information such as a username that the site can retrieve to personalize the page when the user visits the website next time. A cookie can only be re 5 min read
- PHP | Sessions What is a session? In general, session refers to a frame of communication between two medium. A PHP session is used to store data on a server rather than the computer of the user. Session identifiers or SID is a unique number which is used to identify every user in a session based environment. The S 3 min read
- Implementing callback in PHP In PHP, callback is a function object/reference with type callable. A callback/callable variable can act as a function, object method and a static class method. There are various ways to implement a callback. Some of them are discussed below: Standard callback: In PHP, functions can be called using 3 min read
- PHP | Classes Like C++ and Java, PHP also supports object oriented programming Classes are the blueprints of objects. One of the big differences between functions and classes is that a class contains both data (variables) and functions that form a package called an: ‘object’. Class is a programmer-defined data ty 2 min read
- PHP | Constructors and Destructors Constructors are special member functions for initial settings of newly created object instances from a class, which is the key part of the object-oriented concept in PHP5.Constructors are the very basic building blocks that define the future object and its nature. You can say that the Constructors 7 min read
- PHP | Access Specifiers In the PHP each and every property of a class in must have one of three visibility levels, known as public, private, and protected. Public: Public properties can be accessed by any code, whether that code is inside or outside the class. If a property is declared public, its value can be read or chan 4 min read
- Multiple Inheritance in PHP Multiple Inheritance is the property of the Object Oriented Programming languages in which child class or sub class can inherit the properties of the multiple parent classes or super classes. PHP doesn't support multiple inheritance but by using Interfaces in PHP or using Traits in PHP instead of cl 4 min read
- Abstract Classes in PHP Abstract classes are the classes in which at least one method is abstract. Unlike C++ abstract classes in PHP are declared with the help of abstract keyword. Use of abstract classes are that all base classes implementing this class should give implementation of abstract methods declared in parent cl 2 min read
- PHP | Interface An Interface allows the users to create programs, specifying the public methods that a class must implement, without involving the complexities and details of how the particular methods are implemented. It is generally referred to as the next level of abstraction. It resembles the abstract methods, 3 min read
- Static Function in PHP In certain cases, it is very handy to access methods and properties in terms of a class rather than an object. This can be done with the help of static keyword. Any method declared as static is accessible without the creation of an object. Static functions are associated with the class, not an insta 3 min read
- PHP | Namespace Like C++, PHP Namespaces are the way of encapsulating items so that same names can be reused without name conflicts. It can be seen as an abstract concept in many places. It allows redeclaring the same functions/classes/interfaces/constant functions in the separate namespace without getting the fata 2 min read

MySQL Database
- PHP | MySQL Database Introduction What is MySQL? MySQL is an open-source relational database management system (RDBMS). It is the most popular database system used with PHP. MySQL is developed, distributed, and supported by Oracle Corporation. The data in a MySQL database are stored in tables which consists of columns and rows.MySQL 4 min read
- PHP | MySQL ( Creating Database ) What is a database? Database is a collection of inter-related data which helps in efficient retrieval, insertion and deletion of data from database and organizes the data in the form of tables, views, schemas, reports etc. For Example, university database organizes the data about students, faculty, 3 min read
- PHP Database connection The collection of related data is called a database. XAMPP stands for cross-platform, Apache, MySQL, PHP, and Perl. It is among the simple light-weight local servers for website development. Requirements: XAMPP web server procedure: Start XAMPP server by starting Apache and MySQL. Write PHP script f 2 min read
- Connect PHP to MySQL What is MySQL? MySQL is an open-source relational database management system (RDBMS). It is the most popular database system used with PHP. Structured Query Language (SQL). The data in a MySQL database are stored in tables that consist of columns and rows. MySQL is a database system that runs on a s 3 min read
- PHP | MySQL ( Creating Table ) What is a table? In relational databases, and flat file databases, a table is a set of data elements using a model of vertical columns and horizontal rows, the cell being the unit where a row and column intersect. A table has a specified number of columns, but can have any number of rows. Creating a 3 min read
- PHP | Inserting into MySQL database Inserting data into a MySQL database using PHP is a crucial operation for many web applications. This process allows developers to dynamically manage and store data, whether it be user inputs, content management, or other data-driven tasks. In this article, We will learn about How to Inserting into 6 min read
- PHP | MySQL Select Query The SQL SELECT statement is used to select the records from database tables. Syntax : The basic syntax of the select clause is - To select all columns from the table, the [TEX] * [/TEX] character is used. Implementation of the Select Query : Let us consider the following table ' Data ' with three co 3 min read
- PHP | MySQL Delete Query The DELETE query is used to delete records from a database table. It is generally used along with the "Select" statement to delete only those records that satisfy a specific condition. Syntax : The basic syntax of the Delete Query is - Let us consider the following table "Data" with four columns ' I 2 min read
- PHP | MySQL WHERE Clause The WHERE Clause is used to filter only those records that are fulfilled by a specific condition given by the user. in other words, the SQL WHERE clause is used to restrict the number of rows affected by a SELECT, UPDATE or DELETE query. Syntax : The basic syntax of the where clause is - SELECT Colu 3 min read
- PHP | MySQL UPDATE Query The MySQL UPDATE query is used to update existing records in a table in a MySQL database. It can be used to update one or more field at the same time. It can be used to specify any condition using the WHERE clause. Syntax : The basic syntax of the Update Query is - Implementation of Where Update Que 2 min read
- PHP | MySQL LIMIT Clause In MySQL the LIMIT clause is used with the SELECT statement to restrict the number of rows in the result set. The Limit Clause accepts one or two arguments which are offset and count.The value of both the parameters can be zero or positive integers. Offset:It is used to specify the offset of the fir 3 min read
Complete References
- PHP Array Functions PHP Arrays are a data structure that stores multiple elements of a similar type in a single variable. The arrays are helpful to create a list of elements of similar type. It can be accessed using their index number or key. The array functions are allowed to interact and manipulate the array elements 6 min read
- PHP String Functions Complete Reference Strings are a collection of characters. For example, 'G' is the character and 'GeeksforGeeks' is the string. Installation: These functions are not required any installation. These are the part of PHP core. The complete list of PHP string functions are given below: Example: This program helps us to c 6 min read
- PHP Math Functions Complete Reference The predefined math functions in PHP are used to handle the mathematical operations within the integer and float types. These math functions are part of the PHP core. Installation: These functions have not required any installation. The complete list of PHP math functions are given below: Example: P 3 min read
- PHP Filesystem Functions Complete Reference The Filesystem function is used to access and manipulate filesystem. It is the part of PHP code so no need to install these functions. For accessing the files on the system, the file path will be used. On Unix system, forward slash (/) is used as a directory separator and on Windows platform, both f 4 min read
- PHP intl Functions Complete Reference The Complete list of PHP intl Functions are listed below: Collator PHP collator_asort() FunctionPHP collator_compare() FunctionPHP Collator __construct() FunctionPHP Collator create() FunctionPHP collator_sort_with_sort_keys() FunctionPHP collator_sort() Function IntlCalendar PHP IntlCalendar add() 1 min read
- PHP IntlChar Functions Complete Reference IntlChar() functions provide access of utility methods which is used to access the information about Unicode characters. The IntlChar methods and constants are close to the names and behavior which are used by ICU (International Components for Unicode) library. Example: programs illustrate the IntlC 4 min read
- PHP Image Processing and GD Functions Complete Reference Image processing and GD functions are used to create and manipulate image files in different image formats including GIF, PNG, JPEG, WBMP, and XPM. PHP can deliver the output image directly to the browser. The image processing and GD functions are used to compile the image functions for this work. I 3 min read
- PHP Imagick Functions Complete Reference The Imagick Function is used to create and modify images using the ImageMagick API. ImageMagick is the software suite to create edit and modify the composing bitmap images. Thisread, writes and converts images in many formats including DPX, EXR, GIF, JPEG, JPEG-2000, PDF, PhotoCD, PNG, Postscript, S 6 min read
- PHP ImagickDraw Functions Complete Reference The ImagickDraw class is used to draw the vector-based image using ImageMagick. The vector-based generated image can be saved into the file. Syntax: bool ImagickDraw::s() Example: Below program illustrates the ImagickDraw::point() Function in PHP: C/C++ Code <?php // Create an ImagickDraw object 3 min read
- PHP Gmagick Functions Complete Reference Gmagick is the PHP extension used to create, modify and obtain meta information of an image using GraphicsMagick API. The Gmagick consists of Gmagick, GmagickDraw, and GmagickPixel class. Example: Below programs illustrate the Gmagick::getpackagename()Function in PHP: Original Image: C/C++ Code < 3 min read
- PHP GMP Functions Complete Reference The GMP function uses arbitrary-length integers to perform mathematical operations. The GMP function contains the GMP number as an argument. Example: Program to perform the division of GMP numbers when numeric strings as GMP numbers are passed as arguments. C/C++ Code <?php // PHP program to perf 3 min read
- PHP Ds\Set Functions Complete Reference Set is the collection of unique values. The implementation of Ds\Set is similar to the Ds\Map which creates a hash table. The values of Ds\Set are used as key and the mapped values are ignored. Requirements: PHP 7 is required for both extension and the compatibility polyfill. Installation: The easie 2 min read
- PHP Ds\Map Functions Complete Reference A Map is a sequential collection of key-value pair which is very similar to the array. The key of a map can be of any type and it is unique. If any value added to the same key in a Map then the map value will replace. It is the efficient Data Structure in PHP 7 to provide the alternative of an array 3 min read
- PHP Ds\Stack Functions Complete Reference Stack is a linear data structure that follows a particular order in which the operations are performed. The order may be LIFO(Last In First Out) or FILO(First In Last Out). The Ds\Stack uses Ds\Vector internally. Requirements: PHP 7 is required for both extension and the compatibility polyfill. Inst 2 min read
- PHP Ds\Queue Functions Complete Reference A Queue is a linear data structure that follows a particular order in which the operations are performed. The order of queue is First In First Out (FIFO). Requirements: PHP 7 is required for both extension and the compatibility polyfill. Installation: The easiest way to install data structure by usi 2 min read
- PHP Ds\PriorityQueue Functions Complete Reference PriorityQueue is similar to a Queue data structure. The elements of the priority queue are pushed into the queue with a given priority. The highest priority element will always been present at the front of the queue. The priority queue is implemented using the max heap. Requirements: PHP 7 is requir 2 min read
- PHP Ds\Deque Functions Complete Reference A deque data structure is used to store the sequence of values in a contiguous memory buffer which grows and shrinks automatically. The deque is the abbreviation of "double-ended queue". Requirements: PHP 7 is required for both extension and the compatibility polyfill. Installation: The easiest way 3 min read
- PHP Ds\Sequence Functions Complete Reference A Sequence is used to arrange the values in a single and linear dimension way. In some languages, the sequence refers to the List. The sequence is similar to the array which is used as incremental integer keys are listed below: The index value of the sequence are [0, 1, 2, …, size - 1], where size r 3 min read
- PHP SPL Data structures Complete Reference Standard PHP Library (SPL) the collection of standard data structures. The SPL data structure grouped the contents according to their implementation. Example: Below programs illustrate the SplDoublyLinkedList::offsetGet() function in PHP: C/C++ Code <?php // Declare an empty SplDoublyLinkedList $ 4 min read
- PHP Ds\Vector Functions Complete Reference A Vector Data Structure is used to store the sequence of values in a contiguous memory buffer which grows and shrinks automatically. The vector data structure mapped the index value to its index buffer and the growth factor isn't bound to a specific multiple or exponent. It is the efficient Data Str 3 min read
- PHP DOM Functions Complete Reference PHP DOM extension is used to operate on XML documents using DOM API. The DOM extension uses UTF-8 encoding. The Complete list of PHP DOM Functions are listed below: DOMAttr PHP DOMAttr __construct() FunctionPHP DOMAttr isId() Function DOMCdataSection PHP DOMCdataSection __construct() Function DOMCha 2 min read
- PHP Exercises, Practice Questions and Solutions Are you eager to master PHP or looking to sharpen your skills? Dive into our PHP Exercises, Practice Questions, and Solutions designed for both beginners and experienced developers. With our interactive platform, you can engage in hands-on coding challenges, monitor your progress, and enhance your w 3 min read
- Web Technologies
- PHP-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
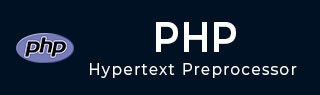
- PHP Developer's AI Tools
- PHP - AI Assistant
- PHP - AI Code Generator
- PHP - AI Code Converter
- PHP - AI Code Debugger
- PHP - AI Code Reviewer
- PHP - AI Code Explainer
- PHP - AI Code Refactoring
- PHP - AI Code Documentation
- PHP - AI Based Interview Preparation
- PHP Tutorial
- PHP - Roadmap
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmetic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook and Paypal Integration
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - Callbacks
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Cheatsheet
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Online Compiler
- PHP - Useful Resources
- PHP - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
PHP - Assignment Operators Examples
You can use assignment operators in PHP to assign values to variables. Assignment operators are shorthand notations to perform arithmetic or other operations while assigning a value to a variable. For instance, the "=" operator assigns the value on the right-hand side to the variable on the left-hand side.
Additionally, there are compound assignment operators like +=, -= , *=, /=, and %= which combine arithmetic operations with assignment. For example, "$x += 5" is a shorthand for "$x = $x + 5", incrementing the value of $x by 5. Assignment operators offer a concise way to update variables based on their current values.
The following table highligts the assignment operators that are supported by PHP −
The following example shows how you can use these assignment operators in PHP −
It will produce the following output −
PHP Assignment Operators
Php tutorial index.
PHP assignment operators applied to assign the result of an expression to a variable. = is a fundamental assignment operator in PHP. It means that the left operand gets set to the value of the assignment expression on the right.
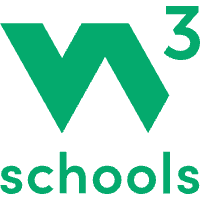
PHP Tutorial
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
PHP Assignment Operators: A Comprehensive Guide for Beginners
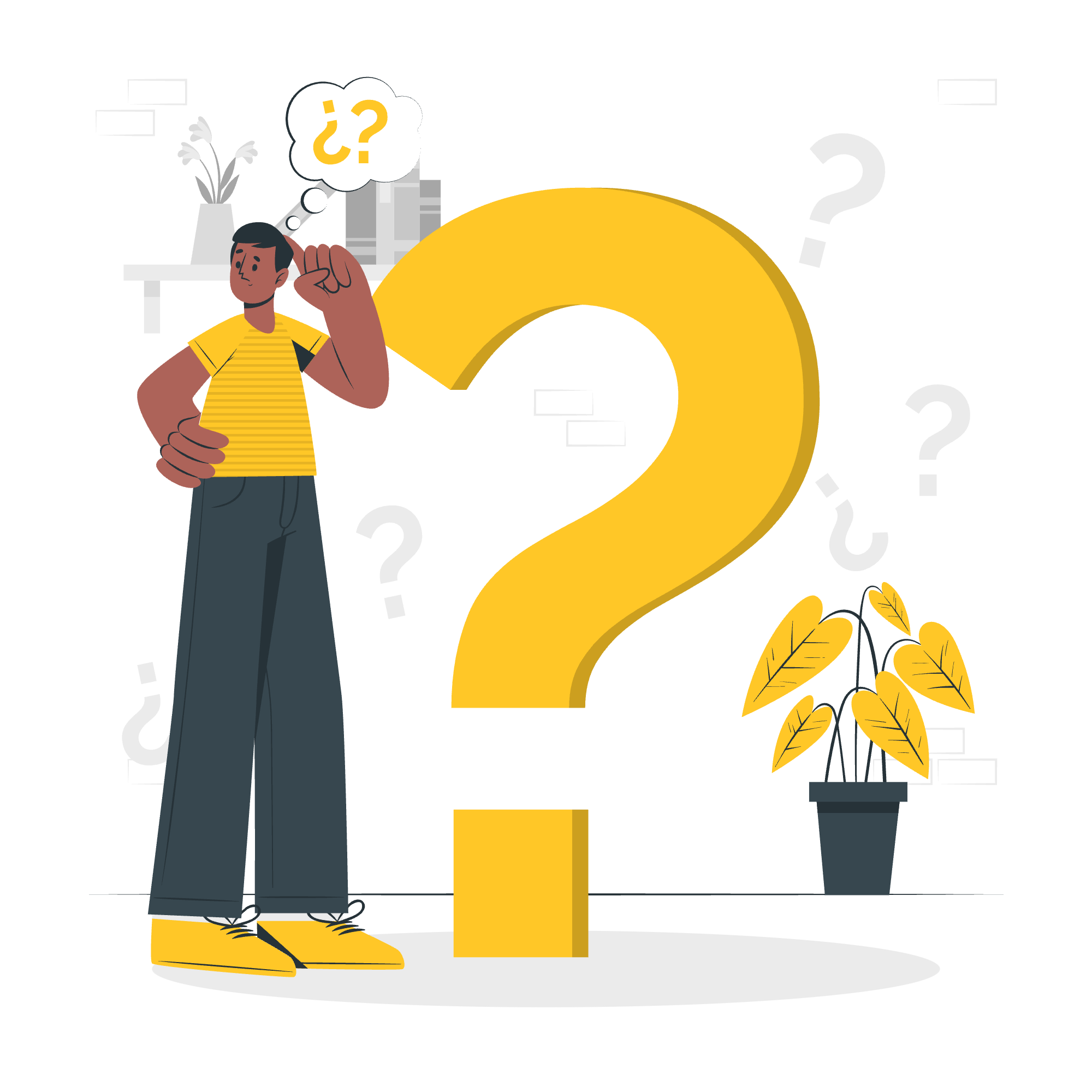
What Are Assignment Operators?
Before we dive into the deep end, let's start with the basics. Assignment operators in PHP are like the helpful assistants in your code kitchen. They take a value and assign it to a variable, much like you'd put ingredients into a mixing bowl. The most common assignment operator is the humble equals sign (=).
The Basic Assignment Operator (=)
Let's look at a simple example:
In this case, we're telling PHP, "Hey, please remember that my_age is 25." It's that simple!
Compound Assignment Operators
Now, let's spice things up a bit. PHP has a set of compound assignment operators that combine an arithmetic operation with assignment. These operators are like kitchen gadgets that chop and mix at the same time – they're real time-savers!
Here's a table of the compound assignment operators in PHP:
Let's break these down with some tasty examples!
The Addition Assignment Operator (+=)
Here, we started with 5 apples and added 3 more. The += operator does this in one swift motion!
The Subtraction Assignment Operator (-=)
Oops! Someone ate 2 cookies. The -= operator helps us keep track of our dwindling cookie supply.
The Multiplication Assignment Operator (*=)
Rabbits multiply fast, don't they? The *= operator helps us model this rapid growth!
The Division Assignment Operator (/=)
Sharing is caring! We've divided our pizza slices by 2, perhaps to share with a friend.
The Modulus Assignment Operator (%=)
This one's a bit tricky. It divides $candies by 5 and gives us the remainder. It's like asking, "If we share 17 candies among 5 people, how many are left over?"
The Exponentiation Assignment Operator (**=)
Bacteria can double every generation. This operator helps us calculate exponential growth easily!
String Concatenation Assignment Operator (.=)
Last but not least, let's talk about the string concatenation assignment operator. It's like a word game where we keep adding new words to make a sentence.
We started with "Hello" and added " World" to it. The .= operator does this joining for us in one step!
Practical Examples
Now that we've covered all the operators, let's put them to use in a fun little program:
This program simulates a simple game where a player's score changes based on various events. Each line demonstrates a different assignment operator in action.
And there you have it, folks! We've journeyed through the land of PHP assignment operators, from the simple = to the more complex compound operators. Remember, these operators are your friends – they're here to make your coding life easier and your programs more efficient.
Practice using these operators in your own code. Try creating a simple calculator or a text-based game. The more you use them, the more natural they'll become.
Happy coding, and may your variables always be correctly assigned!
Credits: Image by storyset
Please wait while your request is being verified...
TutorialKart
- PHP Tutorial
- PHP Install
- PHP Hello World
- Check if variable is set
- Convert string to int
- Convert string to float
- Convert string to boolean
- Convert int to string
- Convert int to float
- Get type of variable
- Subtraction
- Multiplication
- Exponentiation
- Simple Assignment
- Addition Assignment
- Subtraction Assignment
- Multiplication Assignment
- Division Assignment
- Modulus Assignment
- PHP Comments
- Python If AND
- Python If OR
- Python If NOT
- Nested foreach
- echo() vs print()
- printf() vs sprintf()
- PHP Strings
- Create a string
- Create empty string
- Compare strings
- Count number of words in string
- Get ASCII value of a character
- Iterate over characters of a string
- Iterate over words of a string
- Print string to console
- String length
- Substring of a string
- Check if string is empty
- Check if strings are equal
- Check if strings are equal ignoring case
- Check if string contains specified substring
- Check if string starts with specific substring
- Check if string ends with specific substring
- Check if string starts with specific character
- Check if string starts with http
- Check if string starts with a number
- Check if string starts with an uppercase
- Check if string starts with a lowercase
- Check if string ends with a punctuation
- Check if string ends with forward slash (/) character
- Check if string contains number(s)
- Check if string contains only alphabets
- Check if string contains only numeric digits
- Check if string contains only lowercase
- Check if string contains only uppercase
- Check if string value is a valid number
- Check if string is a float value
- Append a string with suffix string
- Prepend a string with prefix string
- Concatenate strings
- Concatenate string and number
- Insert character at specific index in string
- Insert substring at specific index in string
- Repeat string for N times
- Replace substring
- Replace first occurrence
- Replace last occurrence
- Trim spaces from edges of string
- Trim specific characters from edges of the string
- Split string
- Split string into words
- Split string by comma
- Split string by single space
- Split string by new line
- Split string by any whitespace character
- Split string by one or more whitespace characters
- Split string into substrings of specified length
- Transformations
- Reverse a string
- Convert string to lowercase
- Convert string to uppercase
- Join elements of string array with separator string
- Delete first character of string
- Delete last character of string
- Count number of occurrences of substring in a string
- Count alphabet characters in a string
- Find index of substring in a string
- Find index of last occurrence in a string
- Find all the substrings that match a pattern
- Check if entire string matches a regular expression
- Check if string has a match for regular expression
- Sort array of strings
- Sort strings in array based on length
- Foramatting
- PHP – Format string
- PHP – Variable inside a string
- PHP – Parse JSON String
- Conversions
- PHP – Convert CSV String into Array
- PHP – Convert string array to CSV string
- Introduction to Arrays
- Indexed arrays
- Associative arrays
- Multi-dimensional arrays
- String array
- Array length
- Create Arrays
- Create indexed array
- Create associative array
- Create empty array
- Check if array is empty
- Check if specific element is present in array .
- Check if two arrays are equal
- Check if any two adjacent values are same
- Read/Access Operations
- Access array elements using index
- Iterate through array using For loop
- Iterate over key-value pairs using foreach
- Array foreach()
- Get first element in array
- Get last element in array
- Get keys of array
- Get index of a key in array
- Find Operations
- Count occurrences of specific value in array
- Find index of value in array
- Find index of last occurrence of value in array
- Combine two arrays to create an Associative Array
- Convert array to string
- Convert array to CSV string
- Join array elements
- Reverse an array
- Split array into chunks
- Slice array by index
- Slice array by key
- Preserve keys while slicing array
- Truncate array
- Remove duplicate values in array
- Filter elements in array based on a condition
- Filter positive numbers in array
- Filter negative numbers in array
- Remove empty strings in array
- Delete Operations
- Delete specific value from array
- Delete value at specific index in array
- Remove first value in array
- Remove last value in array
- Array - Notice: Undefined offset: 0
- JSON encode
- Array Functions
- array_chunk()
- array_column()
- array_combine()
- array_change_key_case()
- array_count_values()
- array_diff_assoc()
- array_diff_key()
- array_diff_uassoc()
- array_diff_ukey()
- array_diff()
- array_fill()
- array_fill_keys()
- array_flip()
- array_key_exists()
- array_keys()
- array_pop()
- array_product()
- array_push()
- array_rand()
- array_replace()
- array_sum()
- array_values()
- Create an object or instance
- Constructor
- Constants in class
- Read only properties in class
- Encapsulation
- Inheritance
- Define property and method with same name in class
- Uncaught Error: Cannot access protected property
- Multiple catch blocks
- Catch multiple exceptions in a single catch block
- Print exception message
- Throw exception
- User defined Exception
- Get current timestamp
- Get difference between two given dates
- Find number of days between two given dates
- Add specific number of days to given date
PHP Assignment Operators
In this tutorial, you shall learn about Assignment Operators in PHP, different Assignment Operators available in PHP, their symbols, and how to use them in PHP programs, with examples.
Assignment Operators
Assignment Operators are used to perform to assign a value or modified value to a variable.
Assignment Operators Table
The following table lists out all the assignment operators in PHP programming.
In the following program, we will take values in variables $x and $y , and perform assignment operations on these values using PHP Assignment Operators.
PHP Program
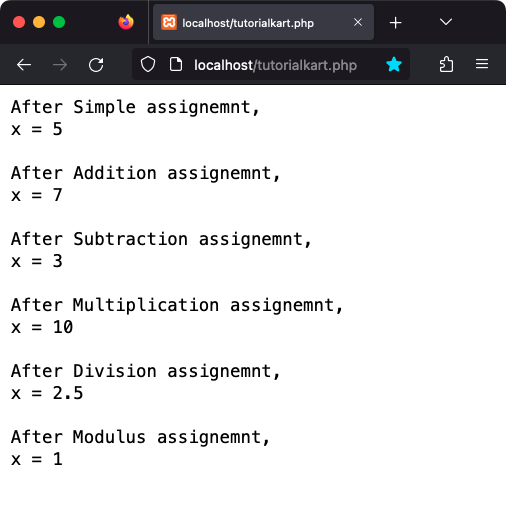
Assignment Operators Tutorials
The following tutorials cover each of the Assignment Operators in PHP in detail with examples.
- PHP Simple Assignment
- PHP Addition Assignment
- PHP Subtraction Assignment
- PHP Multiplication Assignment
- PHP Division Assignment
- PHP Modulus Assignment
In this PHP Tutorial , we learned about all the Assignment Operators in PHP programming, with examples.
Popular Courses
- Salesforce Admin
- Salesforce Developer
- Visualforce
- Informatica
SAP Resources
- Kafka Tutorial
- Spark Tutorial
- Tomcat Tutorial
- Python Tkinter
Programming
- Android Compose
- Kotlin Android
- Bash Script
Web & Server
- Selenium Java
- Definitions
- General Knowledge

IMAGES
COMMENTS
Assignment Operators. The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". Don't. It really means that the left operand gets set to the value of the expression on the right (that is, "gets set to").
Use PHP assignment operator (=) to assign a value to a variable. The assignment expression returns the value assigned. The assignment expression returns the value assigned. Use arithmetic assignment operators to carry arithmetic operations and assign at the same time.
PHP Assignment Operators. The PHP assignment operators are used with numeric values to write a value to a variable. The basic assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right.
Jun 5, 2024 · Assignment Operators: These operators are used to assign values to different variables, with or without mid-operations. Here are the assignment operators along with their syntax and operations, that PHP provides for the operations.
PHP - Assignment Operators Examples - You can use assignment operators in PHP to assign values to variables. Assignment operators are shorthand notations to perform arithmetic or other operations while assigning a value to a variable. For instance, the = operator assigns the value on the right-hand side to the variable on the left-han
PHP assignment operators applied to assign the result of an expression to a variable. = is a fundamental assignment operator in PHP. It means that the left operand gets set to the value of the assignment expression on the right.
Compound Assignment Operators. Now, let's spice things up a bit. PHP has a set of compound assignment operators that combine an arithmetic operation with assignment. These operators are like kitchen gadgets that chop and mix at the same time – they're real time-savers! Here's a table of the compound assignment operators in PHP:
Aug 19, 2022 · The first operand must be a variable and the basic assignment operator is "=". The value of an assignment expression is the final value assigned to the variable. In addition to the regular assignment operator "=", several other assignment operators are composites of an operator followed by an equal sign. Interestingly all five arithmetic ...
Oct 3, 2024 · The most common assignment operator in PHP is an equal (=) operator that can also be used together with various arithmetic operators. Assignment operators provide a compact and efficient way to write complex expressions. We can categorize assignment operators into two types. Basic assignment operator; Compound assignment operators
In this tutorial, you shall learn about Assignment Operators in PHP, different Assignment Operators available in PHP, their symbols, and how to use them in PHP programs, with examples. Assignment Operators. Assignment Operators are used to perform to assign a value or modified value to a variable. Assignment Operators Table